- Feb 22, 2022
- 4 min
Inheritance in Headless Magnolia
Magnolia in action
Take 12 minutes and a coffee break to discover how Magnolia can elevate your digital experience.
A common requirement of many Magnolia projects is for multiple pages of a website to have a consistent structure and look, for example, using the same header and footer. Magnolia’s server side rendering solves this through component inheritance, which means that a page can use components from its parent. Inheritance displays content consistently on multiple pages across the site. When you update content on one page, every page in the page hierarchy displays the same updated content, saving editors the time and effort to configure this manually.
While Magnolia’s SPA renderer and Delivery API do not support component inheritance yet, you can easily build this behaviour into your code. I will show you how to do this using React as an example but the steps are very similar in Angular and Vue.
Configuring the Delivery API to Provide Area Data
The Delivery API allows you to read any node from JCR. To enable inheritance, enable your endpoint to return area nodes by adding mgnl:area to the nodeTypes property.
File: /spa-lm/restEndpoints/delivery/pages.yaml
1class: info.magnolia.rest.delivery.jcr.v2.JcrDeliveryEndpointDefinition
2workspace: website
3depth: 10
4systemProperties:
5 - mgnl:template
6nodeTypes:
7 - mgnl:page
8 - mgnl:area
Now consider a “SPA Home” page template with the areas “header” and “main”.
File: /spa-lm/templates/pages/Home.yaml
1title: SPA Home
2dialog: spa-lm:pages/Home
3templateScript: /spa-lm/webresources/build/index.html
4renderType: spa
5class: info.magnolia.rendering.spa.renderer.SpaRenderableDefinition
6areas:
7 header:
8 title: Header area
9 availableComponents:
10 text:
11 id: spa-lm:components/Text
12 main:
13 title: Main area
14 availableComponents:
15 text:
16 id: spa-lm:components/Text
17 list:
18 id: spa-lm:components/List
This is what the page looks like:
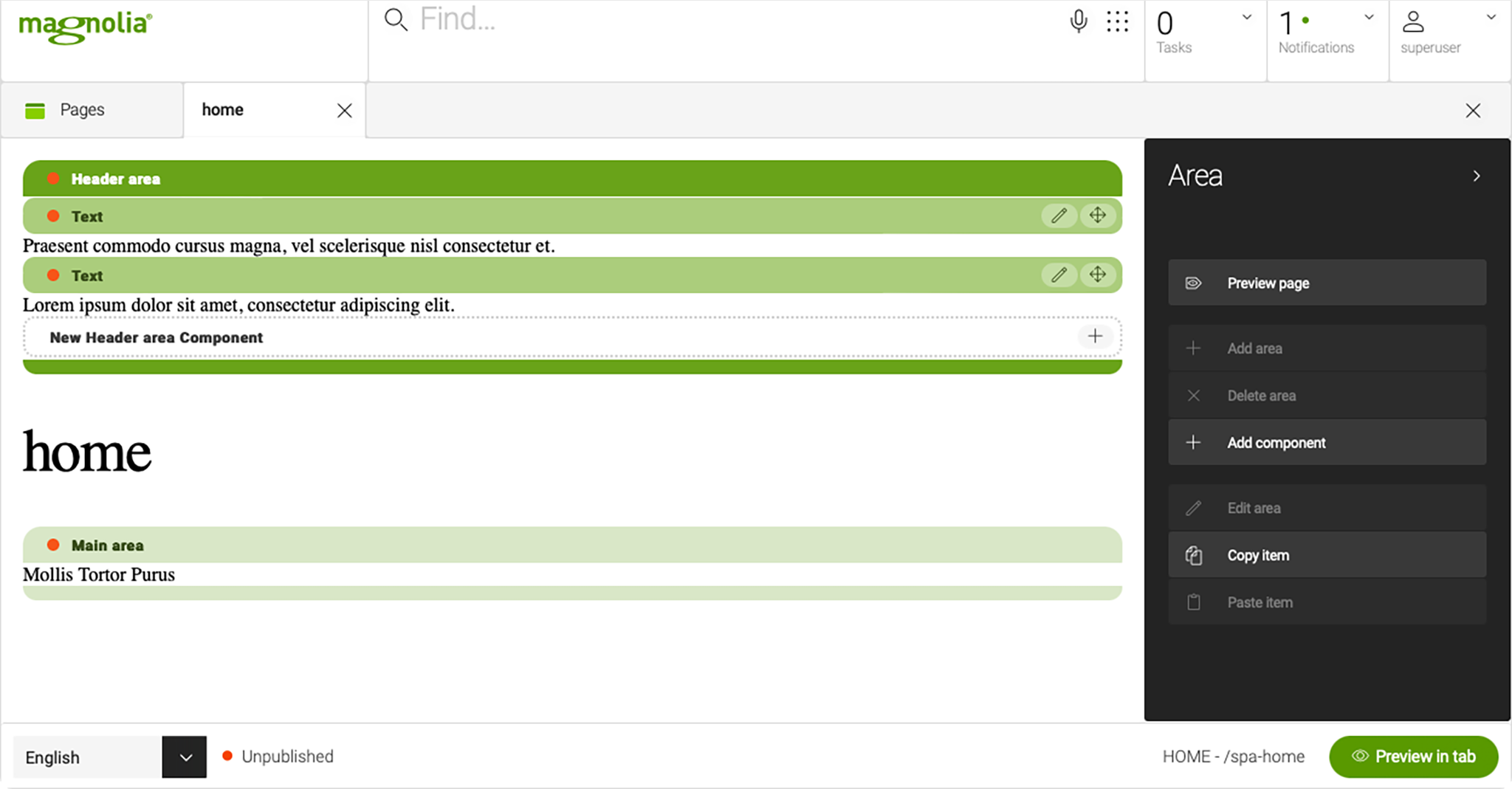
You can now call the pages endpoint to query the “header” area from the home page:
This is what the response should look like:
1{
2 "0": {
3 "@name": "0",
4 "@path": "/spa-home/header/0",
5 "@id": "ae5b767d-a2fb-44fd-8f65-f4c9577ac6eb",
6 "@nodeType": "mgnl:component",
7 "text": "Praesent comodo cursus magna, vet scelerisque nist consectetur et.",
8 "mgnt:template": "spa—tm:components/Text",
9 "@nodes": []
10 },
11 "@name": "header",
12 "@path": "/spa-home/header",
13 "@id": "ae5b767d-a2fb-4ff9-a8dc-4964-97ee-e9cc25c",
14 "@nodeType": "mgnl:area",
15 "00": {
16 "@name": "00",
17 "@path": "/spa-home/header/00",
18 "@id": "768110d6-93e0-466d-98b6-3acac4af4907",
19 "@nodeType": "mgnl:component",
20 "text": "Lorem ipsum dolor sit amet, consectetur adipiscing elit.",
21 "mgnt:template": "spa—tm:components/Text",
22 "@nodes": []
23 },
24 "@nodes": [
25 "0", "00"
26 ]
27}
Headless Magnolia: The Delivery Endpoint API
The Headless Magnolia series explains features that allow you to use Magnolia as a headless CMS. Read our blog post for an introduction to the Delivery endpoint API.
Extending the Page Template
Now, extend the “Home page” template by a function that renders the header’s area only.
1import React, { useState, useEffect } from 'react';
2import { EditableArea } from '@magnolia/react-editor';
3
4export function HomeHeader() {
5 const [header, setHeader] = useState();
6
7 useEffect(() => {
8 async function getHeader() {
9 const res = await fetch('/magnoliaAuthor/.rest/delivery/pages/spa-home/header');
10 const json = await res.json();
11
12 setHeader(json);
13 }
14
15 getHeader();
16 }, []);
17
18 return header ? <EditableArea content={header} /> : null;
19}
20
21function Home(props) {
22 const { title, main, header } = props;
23
24 return (
25 <>
26 {header && <EditableArea content={header} />}
27 <h1>{title}</h1>
28 {main && <EditableArea content={main} />}
29 </>
30 );
31}
32
33export default Home;
The HomeHeader method fetches the header area of the home page and renders it.
Even though we do not use HomeHeader in the “Home page” template (the home page will contain header data and this area can be used directly via props) keeping it close gives clarity and makes it easier to follow the code.
Configuring the Child Page Template
To “inherit” the header area from the home page, the child page needs to make use of the HomeHeader component:
1import React from 'react';
2import { EditableArea } from '@magnolia/react-editor';
3import { HomeHeader } from './Home';
4
5function Contact(props) {
6 const { title, main } = props;
7
8 return (
9 <>
10 <HomeHeader />
11 <h1>{title}</h1>
12 {main && <EditableArea content={main} />}
13 </>
14 );
15}
16
17export default Contact;
When the child page renders the HomeHeader component, it fetches the header data from the parent page. The Magnolia SPA Editor renders the component but does not allow for it to be edited on the child page. To edit the component, it has to be edited on the parent page.
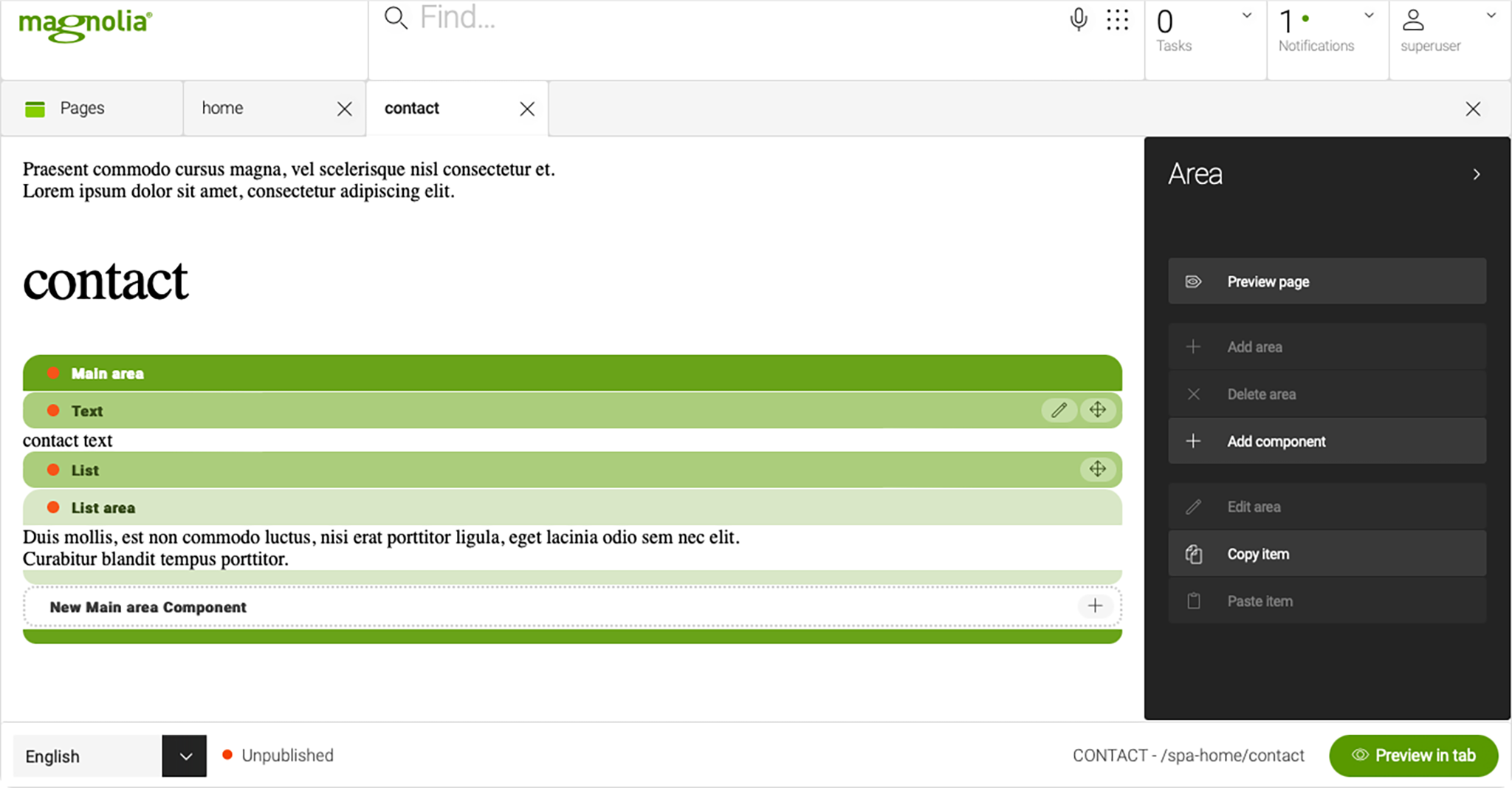
Inheritance Without Inheritance
This simple example shows that we can easily bring inheritance as we know it from FreeMarker rendering into the world of SPA development. It requires a bit of extra code but the results and authoring experience are worth the extra effort.
You can find the entire example including the Magnolia Light Module and SPA code in my repository.
For more information and examples of using Magnolia’s Visual SPA Editor, please check our documentation for your front-end framework:
Learn more about Magnolia’s headless features
This Headless Magnolia series will cover the following topics:
Nodes Endpoint API and Commands Endpoint API
Inheritance in Headless Magnolia (you are here)